When you’re building web applications, being able to handle dates and times efficiently is crucial. Luckily, PHP comes with a powerful set of functions that make working with dates and times a breeze. Whether you need to display the current date, calculate time differences, or format dates in a user-friendly way, PHP has got your back.
In this guide, we’ll dive into the most commonly used PHP date and time functions and walk through some straightforward examples to help you get started.
Getting the Current Date and Time in PHP
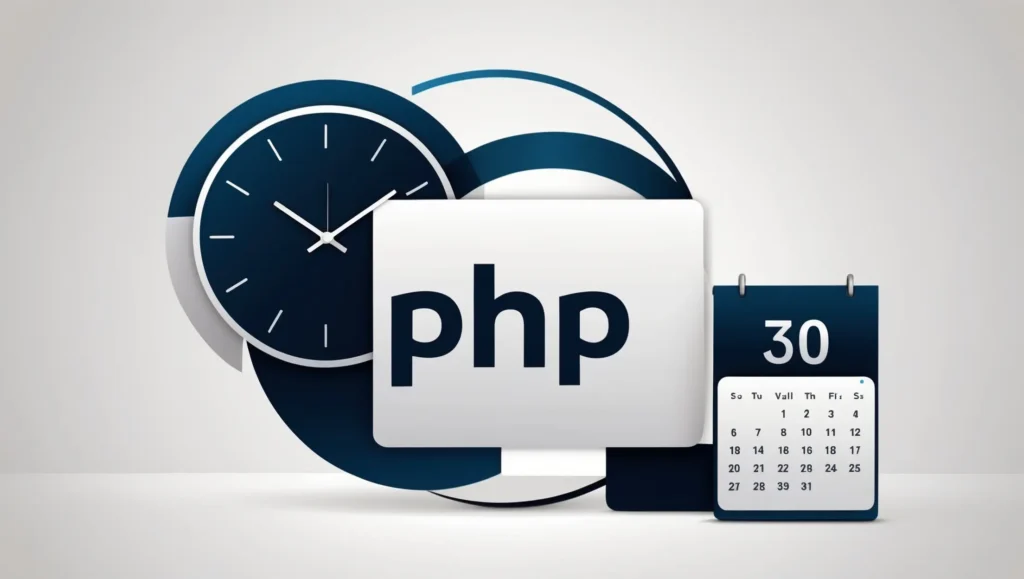
To get the current date and time, you can use the date() function. This function takes a format string and returns the current date and time in that format.
Here’s the basic usage:
echo date('Y-m-d'); // Output: 2024-07-28
echo date('H:i:s'); // Output: 14:25:00 (or the current time)
- ‘Y-m-d’ gives you the date in the format YYYY-MM-DD.
- ‘H:i:s’ provides the time in Hour:Minute:Second format.
You can also customize the output to include other date and time components:
echo date('l, F j, Y'); // Output: Sunday, July 28, 2024
echo date('g:i A'); // Output: 2:25 PM
- ‘l’ gives the full textual representation of the day of the week.
- ‘F j, Y’ gives the full month name, day of the month, and year.
- ‘g:i A’ formats the time in 12-hour format with an AM/PM indicator.
How to work with Timestamps in PHP?
PHP uses Unix timestamps to represent dates and times. A Unix timestamp is a number representing the number of seconds since January 1, 1970.
To get the current timestamp, you can use the time() function:
echo time(); // Output: 1716588000 (an integer representing the current timestamp)
To convert a timestamp back to a human-readable date, use the date() function with the timestamp as a second argument:
$timestamp = time();
echo date('Y-m-d H:i:s', $timestamp); // Output: 2024-07-28 14:25:00
Adding and Subtracting Dates
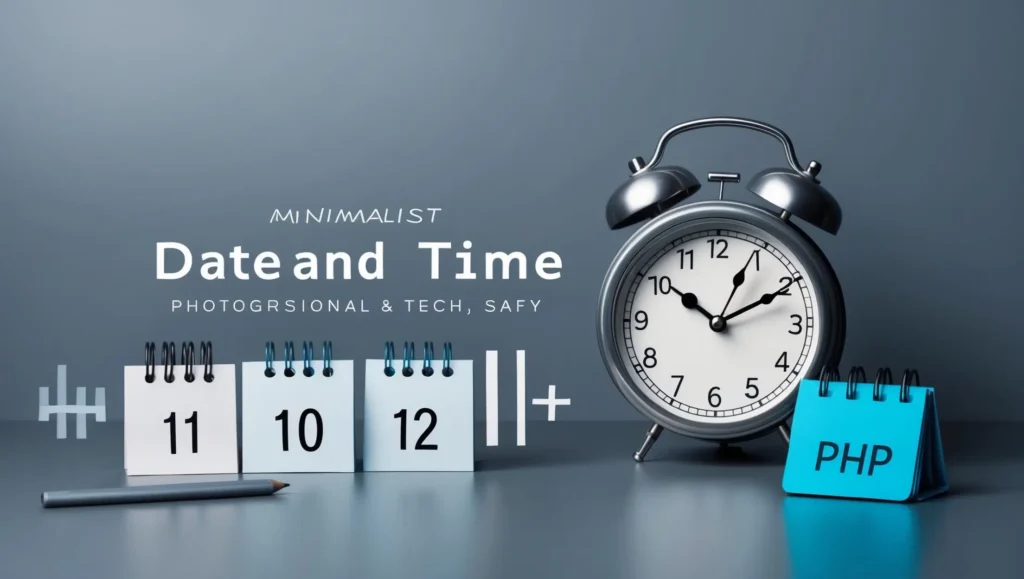
PHP makes it easy to perform date arithmetic using the strtotime() function, which parses a time string into a Unix timestamp.
$tomorrow = strtotime('+1 day');
echo date('Y-m-d', $tomorrow); // Output: 2024-07-29
$yesterday = strtotime('-1 day');
echo date('Y-m-d', $yesterday); // Output: 2024-07-27
How to calculate time differences in PHP?
To calculate the difference between two dates, you can use DateTime objects, which offer a powerful and flexible way to handle date and time.
$date1 = new DateTime('2024-07-28');
$date2 = new DateTime('2024-08-15');
$interval = $date1->diff($date2);
echo $interval->format('%a days'); // Output: 18 days
Working with Timezones in PHP
PHP allows you to handle different timezones with the DateTimeZone class. This can be useful for applications that need to support multiple timezones.
Here’s how you can set a timezone in PHP:
date_default_timezone_set('America/New_York');
echo date('Y-m-d H:i:s');
This tells PHP to use the New York timezone for all date and time operations. You can also create a DateTime object with a specific timezone:
$timezone = new DateTimeZone('Europe/London');
$date = new DateTime('now', $timezone);
echo $date->format('Y-m-d H:i:s');
Conclusion:
PHP’s date and time functions are powerful tools for managing dates and times in your applications. Whether you’re formatting dates, calculating differences, or working with timezones, understanding these functions will help you handle date and time data with ease.
By leveraging the examples provided in this guide, you can start integrating date and time functionality into your PHP projects right away. Happy coding, and may your timezones always be accurate!
FAQ
How to select date and time in PHP?
Use the date() function to select date and time in PHP. like for example: echo date(‘Y-m-d H:i:s’); // Outputs: 2024-07-28 14:30:00
How to set time in 24 hour format in PHP?
Use the date() function with the H:i:s format specifier to set time in 24-hour format in PHP. like for example: echo date(‘H:i:s’); // Outputs: 14:30:00
What is the date_time_set function in PHP?
The date_time_set() function sets the date and time for a DateTime object. Like for example: $datetime = new DateTime(); $datetime->setTime(15, 30, 00); echo $datetime->format(‘Y-m-d H:i:s’); // Outputs: 2024-07-28 15:30:00