PHP, a popular server-side scripting language, offers a rich set of string functions that are essential for handling and manipulating text. In this post, we’ll explore some of the most commonly used PHP string functions, along with brief explanations and code examples to demonstrate their usage.
click this link, if want to learn how to install PHP 8.3
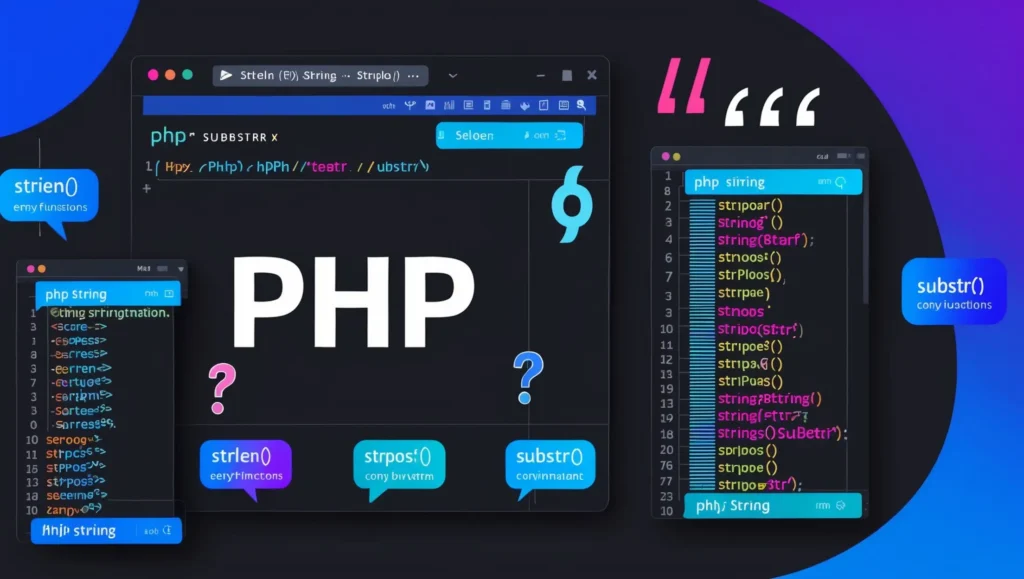
1. `strlen()`: Get String Length
The `strlen()` function returns the length of a string, measured in bytes. This function is useful for determining the size of a string.
Example:
$text = "Hello, World!";
$length = strlen($text);
echo "The length of the string is: $length"; // Outputs: The length of the string is: 13
?>
2. `strpos()`: Find the Position of the First Occurrence of a Substring
The `strpos()` function returns the position of the first occurrence of a specified substring in a string. If the substring is not found, it returns `false`.
Example:
$text = "Hello, World!";
$position = strpos($text, "World");
echo "The position of 'World' is: $position"; // Outputs: The position of 'World' is: 7
?>
3. `substr()`: Extract a Substring
The `substr()` function returns a part of a string, starting from a specified position and optionally up to a specified length.
Example:
$text = "Hello, World!";
$substring = substr($text, 7, 5);
echo "Extracted substring: $substring"; // Outputs: Extracted substring: World
?>
4. `str_replace()`: Replace All Occurrences of a Search String with a Replacement String
The `str_replace()` function replaces all occurrences of a search string within a string with another string.
Example:
$text = "Hello, World!";
$modifiedText = str_replace("World", "PHP", $text);
echo "Modified string: $modifiedText"; // Outputs: Modified string: Hello, PHP!
?>
5. `strtolower()` and `strtoupper()`: Convert String Case
These functions are used to convert a string to lowercase (`strtolower()`) or uppercase (`strtoupper()`).
Example:
$text = "Hello, World!";
$lowercaseText = strtolower($text);
$uppercaseText = strtoupper($text);
echo "Lowercase: $lowercaseText\n"; // Outputs: Lowercase: hello, world!
echo "Uppercase: $uppercaseText"; // Outputs: Uppercase: HELLO, WORLD!
?>
6. `trim()`: Strip Whitespace (or Other Characters) from the Beginning and End of a String
The `trim()` function removes whitespace or other predefined characters from both ends of a string.
Example:
$text = " Hello, World! ";
$trimmedText = trim($text);
echo "Trimmed string: '$trimmedText'"; // Outputs: Trimmed string: 'Hello, World!'
?>
7. `explode()` and `implode()`: Split and Join Strings
`explode()` splits a string into an array based on a delimiter, while `implode()` joins array elements into a single string.
Example:
$text = "apple,banana,cherry";
$array = explode(",", $text);
print_r($array); // Outputs: Array ( [0] => apple [1] => banana [2] => cherry )
$joinedString = implode("-", $array);
echo "Joined string: $joinedString"; // Outputs: Joined string: apple-banana-cherry
?>
Conclusion
PHP’s string functions provide a powerful toolkit for manipulating text, making them indispensable in web development. Whether you need to find, replace, trim, or manipulate strings in other ways, PHP has a function to help you get the job done. Experiment with these functions to better understand how they can simplify your coding tasks and improve the efficiency of your applications.